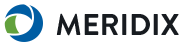 |
LiveID API Version 1.0.18 (23 July 2020) |
Meridix Platform API Documentation
In addition to the docs, we've included code examples, hints, and explanations to help you get started.
The LiveID API uses the REST (REpresentational State Transfer) style with XML responses.
Requirements
1. Register your email address with Meridix, then return here and apply to the Meridix Developer Program.
2. Check LiveID (the organization or team) participation. LiveIDs can choose one of three sets of permissions:
OPEN - which means anyone can access any data in their LiveID (recommended, default)
RESTRICTED - which means the LiveID will only let certain "approved" developers access their data
CLOSED - which means that the LiveID will not let any of their data be accessed via the API
3. Verify that your platform is enabled for sending and receiving XML, and parsing it. For example, we would recommend using PHP with cURL enabled. However, since the API responds with XML, you can use virtually any language or platform that can handle XML responses, such as JavaScript, ASP.NET, Java, C, and many others.
Calling The API
Each time you make a call to the API, you use additional resources. So, the first rule is to construct your code in such a way that you get all the data you need, but you use as few separate requests as you possibly can. Another strategy to help with this is caching, but we'll talk about that at a later time.
First, create a function that you can use which will contain all of your common information, as well as a uniform way that your application or website can connect to the API. For a PHP website, we've created the following include file MakeLiveIDAPICall.php with the MakeLiveIDAPICall function inside of it:
<?php
function MakeLiveIDAPICall($query_string)
{
///// Initialize Your Implementation Variables
$meridixid = "M0000000000";
$api_key = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX";
$base = "https://api.meridix.com/liveid/xml/xml.php";
$url = "$base?meridixid=$meridixid&api_key=$api_key&$query_string";
///// Initialize Your cURL Session
$session = curl_init($url);
///// cURL options are listed at http://us2.php.net/manual/en/function.curl-setopt.php
///// This option tells cURL not to return the headers, but to return the response
curl_setopt($session, CURLOPT_HEADER, false);
///// This option, CURLOPT_RETURNTRANSFER is set to true, cURL stores the response as a variable instead of echoing it.
curl_setopt($session, CURLOPT_RETURNTRANSFER, true);
///// This tells cURL to use HTTP POST
curl_setopt($session, CURLOPT_POST, true);
//send request
$response = curl_exec($session);
//close session
curl_close($session);
//this is the response(xml)
return $response;
}
?>
|
Now that you've got your MakeLiveIDAPICall.php file saved, you can now include it in any php file where you'll be making a call to the API. See this simple example:
<?php
//More verbose error reporting, it may help for debugging
//error_reporting(E_ALL);
//ini_set("display_errors", 1);
//Include the API call function we created earlier
require('MakeLiveIDAPICall.php');
//This array holds one or more parameters, as required by each particular method
$params = array(
'method' => "news.headlines",
'liveid' => "Meridix",
'max' => "15",
);
foreach ($params as $k => $v) {
$query_string .= "$k=" . urlencode($v) . "&";
}
$xml = MakeLiveIDAPICall($query_string);
$response = simplexml_load_string($xml);
if ($response->{'status'} == "OK")
{
//////
////// If the API call was successful, place code here to handle the response
//////
foreach ($response->element as $element)
{
$liveid = $response->{'liveid'};
$eventid = $element->eventid;
$event_type = $element->event_type;
////// Code for handling each instance (an event, a schedule, etc) of data inside the foreach
}
//////
//////
//////
}
else
{
//Some sort of error occurred
print $response->{'method'};
print $response->{'status'};
print $response->{'time'};
print $response->{'meridixid'};
print $response->{'error_type'};
print $response->{'error_reason'};
}
?>
|
Error Handling
If you do happen to encounter any errors, an error response will be returned. Here is an example:
<?xml version="1.0" encoding="UTF-8"?>
<liveid_response>
<method>events.invalidmethod</method>
<status>Error</status>
<time>9/1/2009 11:51:59 PM UTC</time>
<meridixid>M0000000000</meridixid>
<error_type>Method</error_type>
<error_reason>You specified an incorrect request method.</error_reason>
</liveid_response> |
Testing and Debugging
You can use our API XML response testing utility by clicking here. It will display all returned XML from parameters you supply.
Methods and API Documentation
Each API XML response is wrapped in a tag called liveid_response, and will always have the method, status, time, and meridixid information available.
Important Note: The developer meridixid and api_key must always be submitted with every API request.
Available LiveID Methods
Events, Scores, Results
events.live
events.archived
events.quicksummary
Schedule
schedule.details
schedule.quicksummary
schedule.fullsummary
News
news.headlines
news.fullarticle
Photos
photos.quicksummary
Polls
polls.quicksummary
Highlights
highlights.quicksummary
Other Methods Coming Soon
Have a suggested method? Let our developers know
Calls Using Multiple LiveIDs
For those methods which allow more than one LiveID to be specified, use comma delimited format with no spaces for the liveid parameter, such as liveid=MyLiveID1,MyLiveID2,MyLiveID3,MyLiveID4. There is a limit of 20 LiveIDs per API call.
events.live |
|
Method |
events.live |
|
Description |
Returns information on any live event
currently active within one or more LiveIDs. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the live event information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
|
Example Response |
View a live example response using the
API Testing Utility. |
events.archived |
|
Method |
events.archived |
|
Description |
Returns full information about archives
within a particular LiveID or set of LiveIDs, including ability to filter by various
conditions, as well as implement automatic paging. Maximum events returned in a single call
is 1000. If you have a larger set of archives, implement filtering as needed. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the archived event information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- page_number - Optional. Implements optional
paging mechanism for returned results, and this specifies the desired
page number of results being displayed. If implemented, must be
used with the records_per_page parameter. Example:
2
- records_per_page - Optional. Displays the number
of results returned per page, if implemented. Must be used in
conjunction with page_number parameter. Example: 25
- event_type - Optional. This is to filter for
archived events by event type if desired. Example:
Football
- year - Optional. This is to filter by numerical
4-digit year if desired. Example: 2009
- month - Optional. This is to filter by numerical
month if desired. Example: 11
- day - Optional. This is to filter by numerical
day if desired. Example: 27
- keyword - Optional. This is to filter for
archived events by keyword string if desired. Example:
Naperville Central
|
Example Response |
View a live example response using the
API Testing Utility. |
events.quicksummary |
|
Method |
events.quicksummary |
|
Description |
Returns a summary of both live and
archived recent events within a particular LiveID or set of LiveIDs, which is useful for
content areas, tickers, etc. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the recent (both live and archived) event information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- max - Optional. This is the number of events
which you would like returned. If not utilized, list will default
to a maximum of 100. Example: 25
|
Example Response |
View a live example response using the
API Testing Utility. |
schedule.details |
|
Method |
schedule.details |
|
Description |
Returns all information available for a
particular schedule entry. Detailed information includes time,
date, description, and more. |
|
Parameters |
- liveid - Required. This is the LiveID of the
schedule you are accessing. Example:
MyLiveID1
- scheduleid - Required. This is the ID # of the
schedule entry you are obtaining detailed information on.
Example: 1234
|
Example Response |
View a live example response using the
API Testing Utility. |
schedule.quicksummary |
|
Method |
schedule.quicksummary |
|
Description |
Returns a simple summary of scheduled
events for a particular LiveID or set of LiveIDs, which you can then sort by date, event
type, and other parameters. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are obtaining schedule information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- greater_than_date - Optional but highly recommended.
This is to filter for scheduled events that are greater than a certain
date, which is the lower minimum of search range in mm/dd/yyyy format.
Example: 7/21/2009
- less_than_date - Optional but highly recommended.
This is to filter for scheduled events that are less than a certain
date, which represents the upper max of search range in mm/dd/yyyy
format. Example: 12/14/2009
- page_number - Optional. Implements optional
paging mechanism for returned results, and this specifies the desired
page number of results being displayed. If implemented, must be
used with the records_per_page parameter. Example:
2
- records_per_page - Optional. Displays the number
of results returned per page, if implemented. Must be used in
conjunction with page_number parameter. Example: 25
- event_type - Optional. This is to filter for
scheduled events of a certain event type. Example:
Basketball
- event_name - Optional. This is to filter by
keyword in the event name. Example: Naperville
- sort_order - Optional. This is to sort the list
either forward or in reverse. ASC (ascending) or DESC (descending)
only. Example: ASC
|
Example Response |
View a live example response using the
API Testing Utility. |
schedule.fullsummary |
|
Method |
schedule.fullsummary |
|
Description |
Use schedule.quicksummary above
whenever possible because this method, schedule.fullsummary, often
returns more data than is used. Returns a full detailed summary of
scheduled events for a particular LiveID or set of LiveIDs, which you can then sort by
date, event type, and other parameters. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are obtaining schedule information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- greater_than_date - Optional but highly recommended.
This is to filter for scheduled events that are greater than a certain
date, which is the lower minimum of search range in mm/dd/yyyy format.
Example: 7/21/2009
- less_than_date - Optional but highly recommended.
This is to filter for scheduled events that are less than a certain
date, which represents the upper max of search range in mm/dd/yyyy
format. Example: 12/14/2009
- page_number - Optional. Implements optional
paging mechanism for returned results, and this specifies the desired
page number of results being displayed. If implemented, must be
used with the records_per_page parameter. Example:
2
- records_per_page - Optional. Displays the number
of results returned per page, if implemented. Must be used in
conjunction with page_number parameter. Example: 25
- event_type - Optional. This is to filter for
scheduled events of a certain event type. Example:
Basketball
- event_name - Optional. This is to filter by
keyword in the event name. Example: Naperville
- sort_order - Optional. This is to sort the list
either forward or in reverse. ASC (ascending) or DESC (descending)
only. Example: DESC
|
Example Response |
View a live example response using the
API Testing Utility. |
news.headlines |
|
Method |
news.headlines |
|
Description |
Returns a set of the latest news
headlines from a particular LiveID or set of LiveIDs with relevant accompanying information including title,
author, date, story ID number, and more. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the news headlines. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- max - Optional. This is the maximum number of
news items which you would like returned. If not utilized, list
will default to a maximum of 25. Example: 10
|
Example Response |
View a live example response using the
API Testing Utility. |
news.fullarticle |
|
Method |
news.fullarticle |
|
Description |
Returns all available information on a
particular news article including title, author, date, photo URL, story
ID number, and more. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the full article details. Example:
MyLiveID1
- newsid - Required. This is the ID number of the
news article that you are requesting detailed information about.
Example: 1234
|
Example Response |
View a live example response using the
API Testing Utility. |
photos.quicksummary |
|
Method |
photos.quicksummary |
|
Description |
Returns a list of galleries from a LiveID or set of LiveIDs, each with a
set of photos, URLs, and event names, for displaying photo gallery
previews. Launch any gallery given below to
http://www.meridix.com/mbp/photos/photo_viewer.php?GalleryID= [ the
gallery ID here ] in a 790 x 580 window or larger. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting photo information. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- max_galleries - Optional, but highly recommended.
This is the maximum number of total galleries to return. If not
utilized, defaults to 50. Example: 30
- max_photos - Optional, but highly recommended.
This is the maximum number of total photos to return for each gallery.
If not utilized, defaults to 7. Example: 5
|
Example Response |
View a live example response using the
API Testing Utility. |
custom.page |
|
Method |
custom.page |
|
Description |
Returns raw data from custom pages
created by a LiveID, including HTML and other code. |
|
Parameters |
- liveid - Required. This is the LiveID which
created the custom page you are requesting. Example:
MyLiveID1
- pageid - Required.
This is the ID number of the custom page data you are requesting. Example: 1529
|
Example Response |
View a live example response using the
API Testing Utility. |
polls.quicksummary |
|
Method |
polls.quicksummary |
|
Description |
Returns a list of active polls for a
particular LiveID or set of LiveIDs, including poll name, current results, voting options,
and more. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting a list of polls. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
|
Example Response |
View a live example response using the
API Testing Utility. |
highlights.quicksummary |
|
Method |
highlights.quicksummary |
|
Description |
Returns a list of the latest highlights from a particular LiveID or set of LiveIDs, with relevant accompanying information including title,
description, and upload date. |
|
Parameters |
- liveid - Required. This is the LiveID from which
you are requesting the news headlines. Example:
MyLiveID1 or MyLiveID1,MyLiveID2,MyLiveID3
- max - Optional. This is the maximum number of
highlights which you would like returned. If not utilized, list
will default to a maximum of 25. Example: 10
|
Example Response |
View a live example response using the
API Testing Utility. |
Additional Content
Event Link (Shortened Format)
Share a broadcast through social media or anywhere else, using our shortened URL format. Once an event is generated, the link remains the same through its entire lifecycle, whether the event is scheduled, live, or archived.
https://webca.st/[EventID number] such as https://webca.st/62583
Current Live Broadcast Link
Launch the latest live event for a channel, without specifically knowing the event type or EventID number.
https://www.meridix.com/live.php?liveid=[Your LiveID Here] such as https://www.meridix.com/live.php?liveid=Meridix
Channel Home Page Link
Link directly to a channel home page, the starting point for accessing all of a channel's content.
https://www.meridix.com/channel/?liveid=[Your LiveID Here] such as https://www.meridix.com/channel/?liveid=Meridix
RSS News Feeds
By simply adding a news feed URL to a news aggregator (or easy utilities already in most browsers), readers are automatically notified whenever news changes or new stories are posted.
RSS feeds are automatically generated for every LiveID whenever they publish news. Use this URL with a LiveID:
http://www.meridix.com/rss/[Your LiveID In Lowercase Here].xml such as http://www.meridix.com/rss/meridix.xml
© 2025 Meridix API Home Meridix.com Home |